A Guide to Unit Testing with Microsoft Fakes
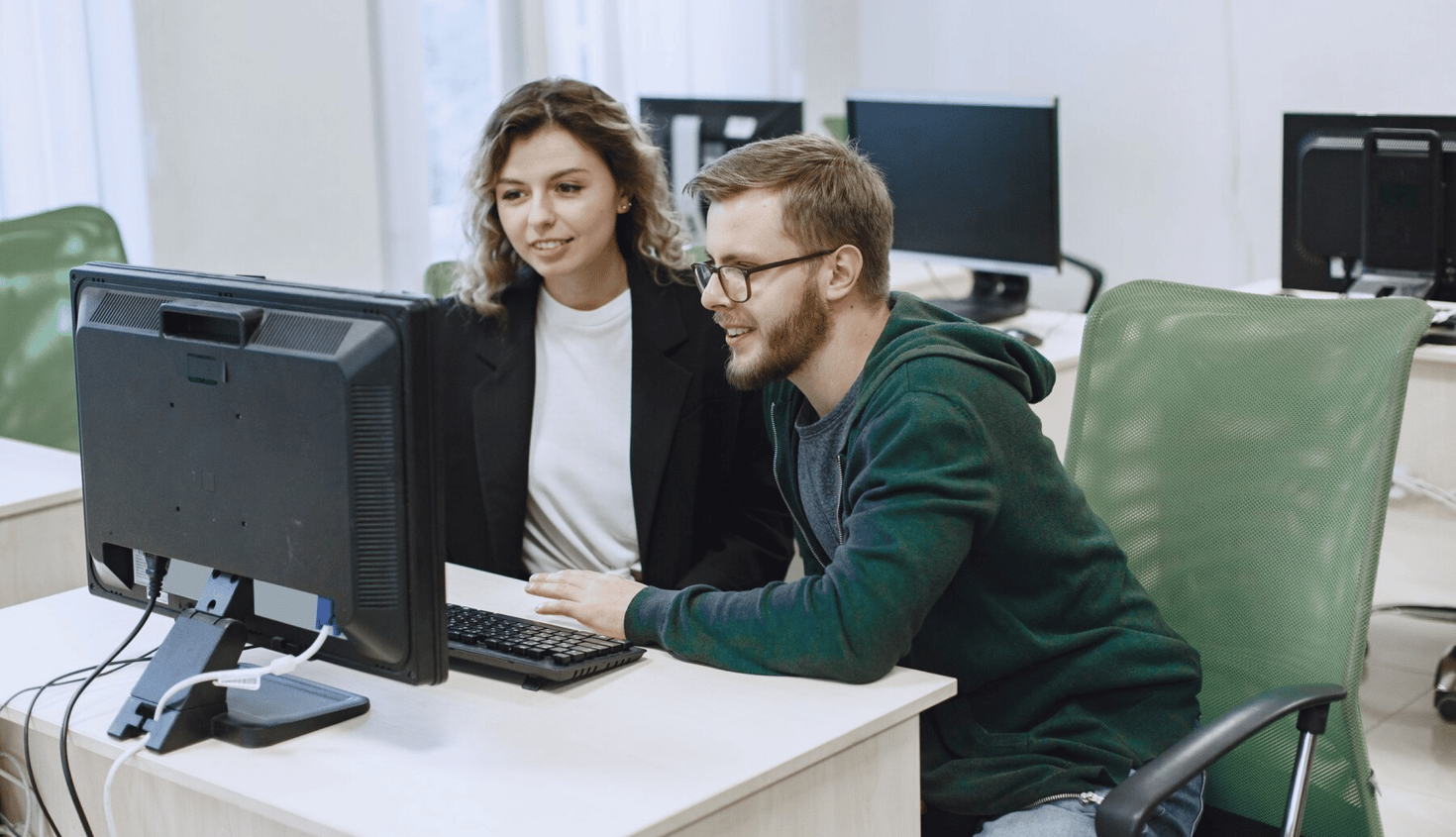
When choosing a mocking framework for your unit tests, you may find quite many options: Moq, Rhino Mocks, NMock, EasyMock.NET, etc. All of them have the similar functionality and are open source.
However, if you or your client prefer commercial products, (e.g. due to better support and guarantees), there is one framework that deserves your interest. It’s Microsoft Fakes. It is available with Visual Studio Premium or Ultimate (starting with 2012). The distinguishing feature of this framework is a replacement of calls to assemblies that you cannot modify. For instance, you can mock System.DateTime.UtcNow of System.dll, which isn’t possible with other mocking frameworks.
In this post, I’ll tell you how you can use this framework to simplify mocking external modules in your unit tests.
Code to be tested
Let’s say we have the following scenario:
- DataProvider generates some data
- LogWriter creates a log from this data and sends it to FileWriter
- FileWriter write this log on the disc
The base entity in our project is Log:

I’m going to make a unit test for LogWriter. It’s inherited form ILogWritter interface:

Log writer is implemented this way:

It has dependencies on IDataProvider and IFileWriter. Their implementation isn’t interesting for us, so I give you only interfaces:

Unit testing
Let’s get started with unit testing! We’ll try to write a unit test for LogWriter:

As you can see, LogWriter constructor requires instances of IDataProvider and IFileWriter. Let’s mock them both. We’ll use Microsoft Fakes Framework for this purpose.
The first step is adding Fakes Assembly
- Open references of your Unit Test Project in solution explorer
- Right click on the assembly that you want to mock
- Select “Add Fakes Assembly”

After these steps, new reference has been added:

Let’s use this Fakes Assembly to mock IDataProvider and IFileWriter.

Fakes assembly provides Stub and Shim for each class. Stubs require that you declare your objects using interfaces rather than classes. It doesn’t allow you to mock a variety of class features like static members. But they give better performance than Shims. Shims are more flexible and work with any class members.
The one important thing that we missed is CreatedDate testing:

The problem with CreatedDate is that we can’t set it from outside. We need to mock System.DateTime.UtcNow in some way. Let’s create Fake System.dll (as I have already mentioned, you cannot do that with any other mocking framework) and make it work as we need.


Now all pieces of code are under control.
Conclusions
As can you see Microsoft Fakes Framework gives us a lot of benefits. The most significant of them are the following:
- You don’t need to find and install any additional packages for mocking
- You can easily replace calls to any assembly (core .Net assemblies for instance)
- It is commercial, so you can count on support.
You could face some difficulties during configuration or running your tests in Continues Integration environment. Here is a hint how to avoid that.
More information can be found here
You can also download the source code from the article here
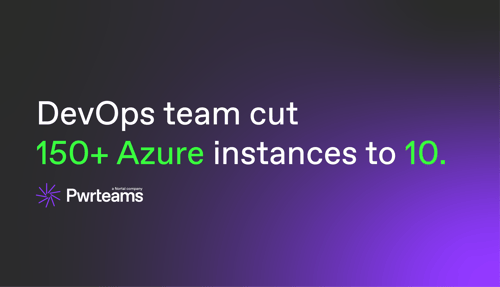
Massive consolidation: reducing 150+ Azure Front Door instances to 10
Read the post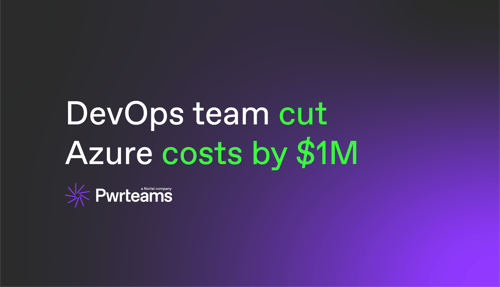
12 months of cloud cost optimisation: how 1 team saved $1M in Azure expenses
Read the post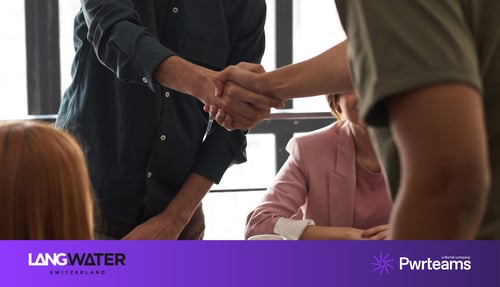
Pwrteams to support the expansion of the Swiss company LANGWATER
Read the postWrite your own
success story
with Pwrteams!
Share your details in the form, tell us about your needs, and we'll get back with the next steps.
- Build a stable team with a 95.7% retention rate.
- Boost project agility and scalability with quality intact.
- Forget lock-ins, exit fees, or volume commitments.